Sign up with SMS by using Amplify Flutter

In the earlier parts of this blog post series, you learned about creating an AWS account, creating a Flutter project and setting up AWS Amplify for your Flutter project.
Now it is time to implement sign up flows!
In the span of this blog post series, you will be learning:
- Creating an AWS Account
- Setting up Amplify CLI on your machine
- Creating a new Flutter project from Flutter CLI tool
- Initializing the AWS Amplify project by using Amplify CLI into your Flutter project
- Implementing a sign up flow with phone number (Current post)
- Implementing a sign in flow with phone number
Sign up users with phone number and email confirmation
In the onPressed
method, you will be calling Amplify.Auth.signUp
method. This will help you to sign up the users but there are some rules to keep in mind:
- Email verification is enabled by default for all cases (unless it is chosen otherwise), therefore you are required to provide an email address in this case
- If you do not want to have email verification, you need to enable phone verification
- Phone number will be passed as username in this case.
Future<void> _signUpUser(String phoneNumber, String password, String email) async {
final result = await Amplify.Auth.signUp(
username: phoneNumber,
password: password,
options: CognitoSignUpOptions(
userAttributes: {
CognitoUserAttributeKey.email: email,
},
),
);
if (result.isSignUpComplete) {
//TODO: Add Sign In flow
debugPrint('Sign up is done.');
} else {
//TODO: Add confirmation flow
debugPrint('Confirmation time! Check your email!');
}
}
The method will check if the users have:
- a phone number as username,
- phone number should have the country code alongside it
- a proper email address,
- a long password (minimum 8 characters)
Otherwise, it will not sign the user up. So it is a good practice to let the user know about these requirements.
When the account needs to be confirmed, users now will be seeing a dialog to enter their confirmation code. The code will be sent to the email address with this iteration.

Now add the confirmation flow:
// Add next to the other controllers at the top of the class
late final TextEditingController _activationCodeController;// Add next to the other controllers at the initState method
_activationCodeController = TextEditingController();// Add next to the other controllers at the dispose method
_activationCodeController.dispose();...showDialog<void>(
context: context,
builder: (context) => AlertDialog(
title: const Text('Confirm the user'),
content: Column(
mainAxisSize: MainAxisSize.min,
children: [
Text('Check your email at $email and enter the code below'),
OutlinedAutomatedNextFocusableTextFormField(
controller: _activationCodeController,
padding: const EdgeInsets.only(top: 16),
labelText: 'Activation Code',
inputType: TextInputType.number,
),
],
),
actions: <Widget>[
TextButton(
child: const Text('Dismiss'),
onPressed: () => Navigator.of(context).pop(),
),
TextButton(
child: const Text('Confirm'),
onPressed: () {
Amplify.Auth.confirmSignUp(
username: phoneNumber,
confirmationCode: _activationCodeController.text,
).then((result) {
if (result.isSignUpComplete) {
Navigator.of(context).pop();
}
});
},
),
],
),
);
Once the code is entered, you can check your user console by writing amplify console auth
to the terminal and see the confirmation status as follows:

Now that you created the full signup flow, it is time to do the confirmation with the phone number!
Sign up users with phone number and phone number confirmation
First of all, start off by removing the email address verification and adding phone number verification:
$ amplify update auth
Using service: Cognito, provided by: awscloudformation
$ What do you want to do?
Apply default configuration with Social Provider (Federation)
❯ Walkthrough all the auth configurations
Create or update Cognito user pool groups
Create or update Admin queries API
$ Select the authentication/authorization services that you want to use: (Use arrow
keys)
❯ User Sign-Up, Sign-In, connected with AWS IAM controls (Enables per-user Storage
features for images or other content, Analytics, and more)
User Sign-Up & Sign-In only (Best used with a cloud API only)
I want to learn more.
$ Allow unauthenticated logins? (Provides scoped down permissions that you can cont
rol via AWS IAM) (Use arrow keys)
Yes
❯ No
I want to learn more.
$ Do you want to enable 3rd party authentication providers in your identity pool?
Yes
❯ No
I want to learn more.
$ Do you want to add User Pool Groups?
Yes
❯ No
I want to learn more.
$ Do you want to add an admin queries API?
Yes
❯ No
I want to learn more.
$ Multifactor authentication (MFA) user login options: (Use arrow keys)
❯ OFF
- ON (Required for all logins, can not be enabled later) (Disabled)
OPTIONAL (Individual users can use MFA)
I want to learn more.
$ Email based user registration/forgot password:
Enabled (Requires per-user email entry at registration)
❯ Disabled (Uses SMS/TOTP as an alternative)$ Please specify an SMS verification message: (Your verification code is {####})$ Do you want to override the default password policy for this User Pool? (y/N)$ Specify the app's refresh token expiration period (in days): (30)$ Do you want to specify the user attributes this app can read and write? (y/N)$ Do you want to enable any of the following capabilities? (Press <space> to select,
<a> to toggle all, <i> to invert selection)
❯◯ Add Google reCaptcha Challenge
◯ Email Verification Link with Redirect
◯ Add User to Group
◯ Email Domain Filtering (denylist)
◯ Email Domain Filtering (allowlist)
◯ Custom Auth Challenge Flow (basic scaffolding - not for production)
◯ Override ID Token Claims
$ Do you want to use an OAuth flow?
Yes
❯ No
I want to learn more.
$ Do you want to configure Lambda Triggers for Cognito? (Y/n)
Now push your changes to the cloud by amplify push
, and go back to your code.
showDialog<void>(
context: context,
builder: (context) => AlertDialog(
title: const Text('Confirm the user'),
content: Column(
mainAxisSize: MainAxisSize.min,
children: [
const Text('Check your phone number and enter the code below'),
OutlinedAutomatedNextFocusableTextFormField(
controller: _activationCodeController,
padding: const EdgeInsets.only(top: 16),
labelText: 'Activation Code',
inputType: TextInputType.number,
),
],
),
actions: <Widget>[
TextButton(
child: const Text('Dismiss'),
onPressed: () => Navigator.of(context).pop(),
),
TextButton(
child: const Text('Confirm'),
onPressed: () {
Amplify.Auth.confirmSignUp(
username: phoneNumber,
confirmationCode: _activationCodeController.text,
).then((result) {
if (result.isSignUpComplete) {
Navigator.of(context).pop();
}
});
},
),
],
),
);
Be mindful that this does not mean you do not need email address. For verifying user information, Amplify either asks for an email address or bunch of personal information e.g. name, surname etc. For the sake of simplicity you will continue with email here.
If you run the application again and register a user, now you will not receive an email. But you will not also receive an SMS yet, because you have not set up a system to send messages yet.
Once you have enabled the SMS based auth workflow, if you looked carefully in your debug console you might remember seeing an error message like below:
⚠️ You have enabled SMS based auth workflow. Verify your SNS account mode in the SNS console: https://console.aws.amazon.com/sns/v3/home#/mobile/text-messaging
If your account is in "Sandbox" mode, you can only send SMS messages to verified recipient phone numbers.
Amplify uses a Simple Notification Service (SNS) for providing message delivery service from publishers to subscribers. For sending SMS messages it is required to have an account on the Amazon SNS.
Registering phone numbers for special request countries
Go to the Amazon SNS messaging page and before you do anything else, check if you have a warning like below at the top of the page:

If you do, this means for some countries you are expected to origination numbers to send the messages, but if you don’t that is good news for you and you can skip to the Registering phone numbers for sandbox destination section.
Now click on the View origination numbers button. It will direct you to the Origination Numbers Console. You can either check the numbers you have there or create a new number by clicking the Provision numbers in Pinpoint button.

That will take you to the Amazon Pinpoint page to add numbers. If your country does not support sender IDs, you must purchase an origination number. In Pinpoint, click on Request phone number. This will bring you to a page where you can obtain a Toll-free number for sending SMS messages. Choose the country from which you’ll be sending SMS messages, then follow the prompts for requesting a new number. After successfully requesting a toll-free number, you can return to SNS to verify your phone number like described at the next section.

Registering Sender IDs for special request countries
If the country you are supporting is not part of the phone numbers list, then you can check the list of countries here to see the requirements. E.g. some countries like Turkey require a sender registration. Senders are required to use a pre-registered alphabetic Sender ID. In SMS messaging, a sender ID is a name that appears as the message sender on recipients’ devices. Support for sender IDs varies by country. For example, carriers in the United States don’t support sender IDs at all, but carriers in India require senders to use sender IDs.
If you need to register a sender ID in India, complete the procedures in Special requirements for India beforeyou open a case in Support Center.
Go to the Service Limit Increase support page:
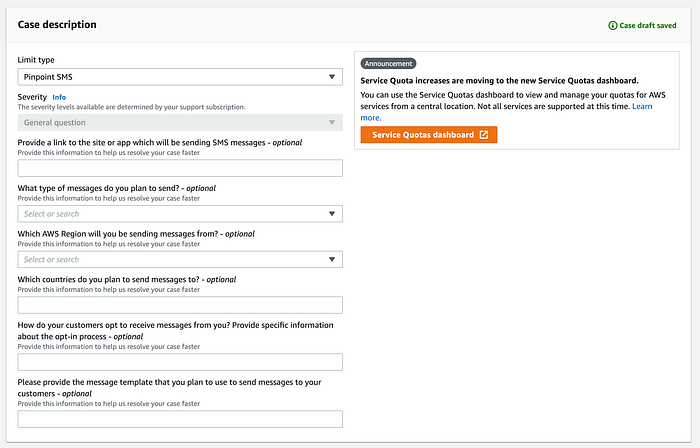
- For Limit type, choose Pinpoint SMS.
- (Optional) For Provide a link to the site or app which will be sending SMS messages, provide information about the website, application, or service that will send SMS messages.
- (Optional) For What type of messages do you plan to send, choose the type of message that you plan to send using your long code:
- One Time Password — Messages that provide passwords that your customers use to authenticate with your website or application.
- Promotional — Noncritical messages that promote your business or service, such as special offers or announcements.
- Transactional — Important informational messages that support customer transactions, such as order confirmations or account alerts. Transactional messages must not contain promotional or marketing content.
- (Optional) For Which AWS Region will you be sending messages from, choose the region that you’ll be sending messages from.
- (Optional) For Which countries do you plan to send messages to, enter the country or region that you want to purchase short codes in.
- (Optional) In the How do your customers opt to receive messages from you, provide details about your opt-in process.
- (Optional) In the Please provide the message template that you plan to use to send messages to your customersfield, include the template that you will be using.
After that, fill in the request information like below (You may pick the Region based on the region you prefer):

Lastly, fill in the case description as the following and submit your request:
Sender id: <SENDER-ID>
SMS Template: <YOUR-MESSAGE> e.g. Your confirmation code is: ####
Planned message count: <ESTIMATED-NUMBER-OF-MESSAGES-TO-SEND>
User opt-in: <TEXT-TO-EXPLAIN-HOW-USERS-OPT-IN>
Organization name: <NAME>
Organization address: <Address>
Organization country: <Country>
Organization phone number: <PHONE-NUMBER>
Organization website: <URL>
After the request is received, an initial response will be provided within 24 hours.
Once the process of obtaining your sender ID is completed, you will be notified. When you receive this notification, complete the steps in this section to configure Amazon Pinpoint to use your sender ID.
- Sign in to the AWS Management Console and open the Amazon Pinpoint console.
- On the All projects page, choose a project that uses the SMS channel.
- In the navigation pane, under Settings, choose SMS and voice.
- Next to SMS settings, choose Edit.
- Under Account-level settings, for Default sender ID, type your sender ID.
- Choose Save changes.
Registering phone numbers for sandbox destination
If your account is in the Sandbox mode, you will be expected to add a phone number and verify it. Go to the Sandbox destination phone number section and click on the Add phone number button.

After that, add a new phone number and verify the number with a message.

After you verify your number and verification status as verified. Now you can send messages from your application. Run your application again and try to create an account. You will now receive a verification code that you can use.

Add it to your confirmation dialog to confirm the user:

Now you should be all set with phone verification!
Next step is wiring up the sign in flow with phone number.
For more information about the AWS Amplify authentication libraries you can check the official documentation, ask your questions at Amplify Discord. You can also check the source code for this over GitHub and if you have any questions regarding to the Amplify and Flutter topics, send it to me via DM on Twitter!
See you in the next post!